andriod service 和intentservice
项目地址:https://gitee.com/vita__chaochao/andriod-backend-app
service和intentservice对比
不同点
service需要主动stop 才会销毁,intentservice程序执行完成后会自动销毁
相同点
等待之前的任务完成后,再执行新的任务,所有任务完成后最后销毁。
1.myservice开发
代码
package com.example.myapplication;
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.app.Service;
import android.content.Intent;
import android.graphics.Color;
import android.os.Build;
import android.os.IBinder;
import android.util.Log;
public class MyService extends Service {
public MyService() {
}
@Override
public IBinder onBind(Intent intent) {
Log.v("Jason", "MyService onBind");
return null;
}
@Override
public void onCreate() {
Log.v("Jason", "MyService onCreate");
super.onCreate();
}
@Override
public void onStart(Intent intent, int startId) {
Log.v("Jason", "MyService onStart");
super.onStart(intent, startId);
}
@Override
public void onDestroy() {
Log.v("Jason", "MyService onDestroy");
super.onDestroy();
}
@Override
public int onStartCommand(Intent intent, int flags, int startId) {
Log.v("Jason", "MyService onStartCommand-----");
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
Log.v("Jason", "MyService onStartCommand after sleep 10 s-----");
//以下为新增---------------------------------------------
String CHANNEL_ONE_ID = "com.primedu.cn";
String CHANNEL_ONE_NAME = "Channel One";
NotificationChannel notificationChannel = null;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
notificationChannel = new NotificationChannel(CHANNEL_ONE_ID,
CHANNEL_ONE_NAME, NotificationManager.IMPORTANCE_HIGH);
notificationChannel.enableLights(true);
notificationChannel.setLightColor(Color.RED);
notificationChannel.setShowBadge(true);
notificationChannel.setLockscreenVisibility(Notification.VISIBILITY_PUBLIC);
NotificationManager manager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
manager.createNotificationChannel(notificationChannel);
}
//--------------------------------------------------------以上为新增
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_IMMUTABLE);
Notification notification = new Notification();
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
notification = new Notification.Builder(this).setChannelId(CHANNEL_ONE_ID)
.setTicker("Nature")
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("xxxx")
.setContentText("setContentText")
.setContentIntent(pendingIntent)
.getNotification();
}
notification.flags |= Notification.FLAG_NO_CLEAR;
startForeground(1, notification);
// service需要手动stop才会销毁
stopSelf(startId);
return super.onStartCommand(intent, flags, startId);
}
}
配置
<service android:name=".MyService"
android:exported="true"
>
</service>
2.intentservice开发
代码
package com.example.myapplication;
import android.app.IntentService;
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Intent;
import android.graphics.Color;
import android.os.Build;
import android.util.Log;
import java.util.Objects;
public class MyIntentService extends IntentService {
/**
* Creates an IntentService. Invoked by your subclass's constructor.
*
* @param name Used to name the worker thread, important only for debugging.
*/
public MyIntentService(String name) {
super(name);
Log.v("Jason", "MyIntentService Creates an IntentService");
}
public MyIntentService() {
super("MyIntentService");
Log.v("Jason", "MyIntentService Creates an IntentService zero argument constructor");
}
@Override
protected void onHandleIntent(Intent intent) {
assert intent != null;
Log.v("Jason", "MyIntentService onHandleWork---------,myvar value is:" + Objects.requireNonNull(intent.getExtras()).getString("myvar"));
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
Log.v("Jason", "MyIntentService onHandleWork after sleep 10s---------" );
//以下为新增---------------------------------------------
String CHANNEL_ONE_ID = "com.primedu.cn";
String CHANNEL_ONE_NAME = "Channel One";
NotificationChannel notificationChannel = null;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
notificationChannel = new NotificationChannel(CHANNEL_ONE_ID,
CHANNEL_ONE_NAME, NotificationManager.IMPORTANCE_HIGH);
notificationChannel.enableLights(true);
notificationChannel.setLightColor(Color.RED);
notificationChannel.setShowBadge(true);
notificationChannel.setLockscreenVisibility(Notification.VISIBILITY_PUBLIC);
NotificationManager manager = (NotificationManager) getSystemService(NOTIFICATION_SERVICE);
manager.createNotificationChannel(notificationChannel);
}
//--------------------------------------------------------以上为新增
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_IMMUTABLE);
Notification notification = new Notification();
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
notification = new Notification.Builder(this).setChannelId(CHANNEL_ONE_ID)
.setTicker("Nature")
.setSmallIcon(R.mipmap.ic_launcher)
.setContentTitle("xxxx")
.setContentText("setContentText")
.setContentIntent(pendingIntent)
.getNotification();
}
notification.flags |= Notification.FLAG_NO_CLEAR;
startForeground(1, notification);
}
@Override
public void onDestroy() {
super.onDestroy();
Log.v("Jason", "MyIntentService onDestroy");
}
}
配置
<!-- start forground需要权限-->
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" />
<service
android:name=".MyIntentService"
android:exported="true">
</service>
3.adb测试
wulili@wulilideMacBook-Air andriod-backend-app % adb shell am start-foreground-service -n "com.example.myapplication/com.example.myapplication.MyService" -e myvar myvalval
Starting service: Intent { cmp=com.example.myapplication/.MyService (has extras) }
wulili@wulilideMacBook-Air andriod-backend-app % adb shell am start-foreground-service -n "com.example.myapplication/com.example.myapplication.MyService" -e myvar myvalval
Starting service: Intent { cmp=com.example.myapplication/.MyService (has extras) }
destroy()最后调用一次
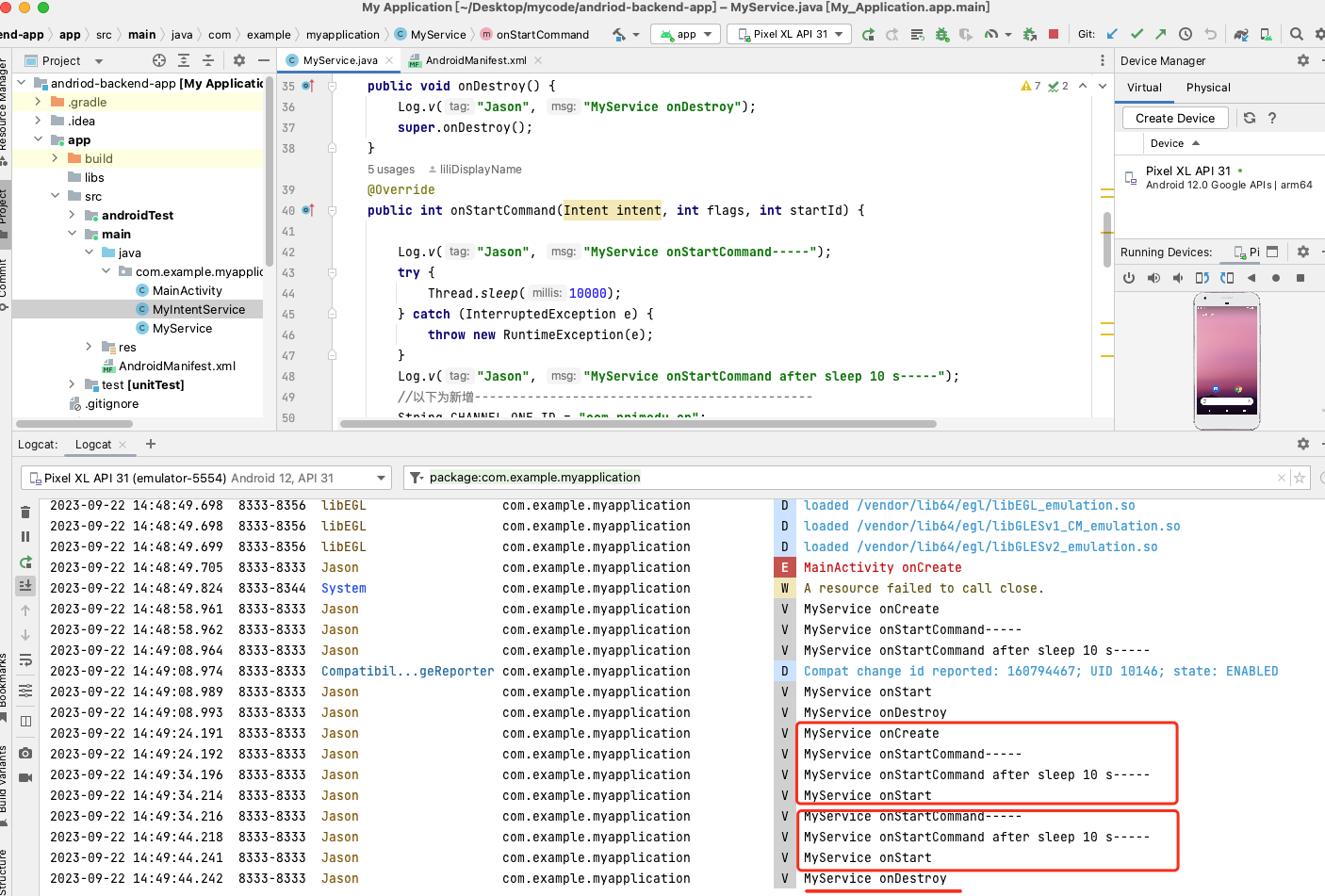
wulili@wulilideMacBook-Air andriod-backend-app % adb shell am start-foreground-service -n "com.example.myapplication/com.example.myapplication.MyIntentService" -e myvar myvalval
Starting service: Intent { cmp=com.example.myapplication/.MyIntentService (has extras) }
wulili@wulilideMacBook-Air andriod-backend-app % adb shell am start-foreground-service -n "com.example.myapplication/com.example.myapplication.MyIntentService" -e myvar myvalval
Starting service: Intent { cmp=com.example.myapplication/.MyIntentService (has extras) }
wulili@wulilideMacBook-Air andriod-backend-app %
destroy()最后调用一次
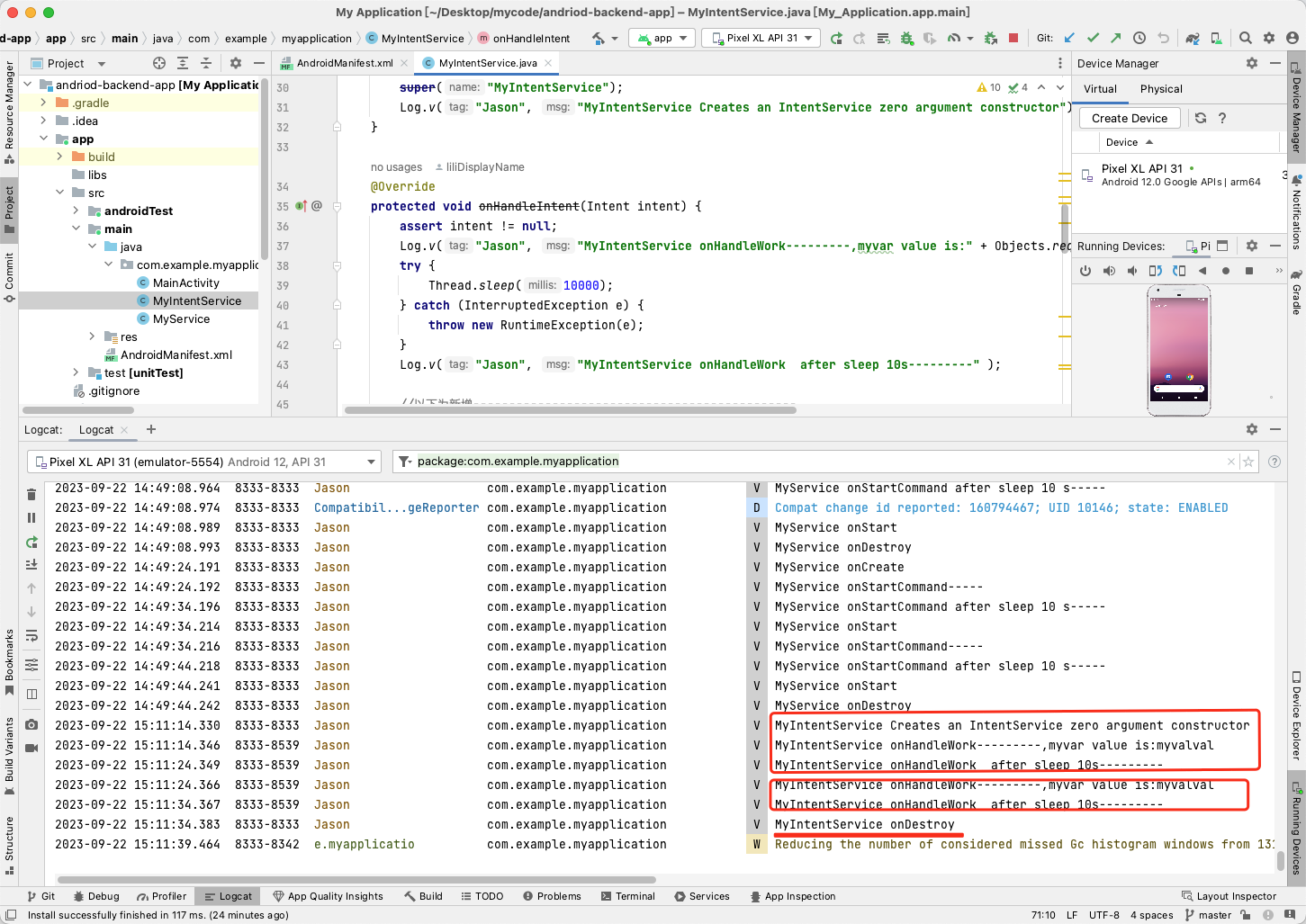
版权声明:原创作品,允许转载,转载时请务必以超链接形式标明文章 原始出处 、作者信息和本声明。否则将追究法律责任。
转载请注明来源:andriod service 和intentservice - 多知在线